Portfolios and Pizza
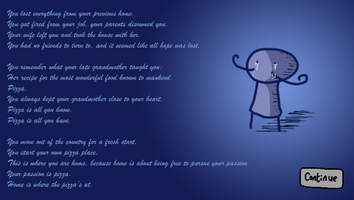
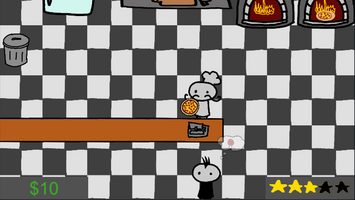
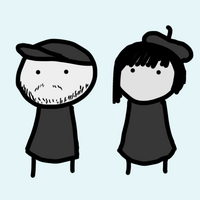
As I continue to work on building out my portfolio, I am revisiting many of my old projects to try and piece them back together into a shareable format. I started working with Unity 3 a bit over a decade ago, and the rapid changes in technology have rendered many of my older projects obsolete. All of my old web games built with the Unity Web Player are no longer supported at all, and if I want them to be playable again, I need to migrate and rebuild them with the "new" HTML5 based WebGL build. However, so many things have changed in the Unity Editor over that course of time, that sometimes just bringing my projects into a newer supported version of the engine breaks nearly everything about them. Unity reworked materials with their new render pipelines after Unity 5, so everything from before then needs complete rebuilds of all materials and shaders. Then there's minor tweaks with other systems like physics, various libraries, and coding practices to contend with as well. (Also, my own programming has improved significantly since many of my earlier projects, so I also find myself needing to improve some of the hackier mistakes I made on older projects).
As an example of one project that needed updating, I just updated and re-released Home Is Where the Pizza's At. First, some background on the game: It was developed in Unity 2019 for the 2019 Global Game Jam. The theme had been "What Home Means To You," and after a brief brainstorming session with my super-talented cousin Mel we settled on "Pizza." Lightly inspired by the mechanics of Overcooked (but deeply simplified for the 48 hour challenge), we built a pizza restaurant simulator with some tragic backstory. We were able to complete the game on time without having to work too unreasonable of hours, and it was generally well-liked.
Home Is Where the Pizza's At was only 4 years old (ok, that does seem like a bit now that I write it down...), but despite that relatively short amount of time, something had changed in the WebGL player and most of the collisions/input were no longer working. I distinctly remembered them working at the time when we released it, so I wasn't quite sure what had happened, so I needed to rebuild it and see if I could fix the issues. I brought it into the latest version of Unity that I've been working with lately (2022.3), and started working through the issues.
Thankfully there weren't too many with this project, but something had definitely changed with the physics. All of the customers entering the shop were incredibly slow, and my inputs for collisions were only sometimes firing. The first problem was a fairly easy fix. I just tweaked the movement speed until I settled on something that worked with the new system, and ended up moving it from 3 to 15, which seemed about the speed I remembered. (They were already being normalized by Time.deltaTime, so it wasn't a framerate issue. I'm just really not sure what happened there). The second problem was a bit more challenging, so I had to come up with a more creative solution.
The original collision methods used the OnTriggerStay2D() event, and then checked Input.GetButtonDown("Jump") (SpaceBar) to run the CheckCollision() function, which would handle all the various object interactions in the game. Something like this:
OnTriggerStay2D(Collider2D collision) { // If SpaceBar is pressed if (Input.GetButtonDown("Jump")) { CheckCollision(collision); } }
This had worked in the old system, but now it was firing intermittently (and mostly not at all). My suspicion is some sort of mismatch between the physics system running on FixedUpdate, and the Input system being frame independent. My best guess is that OnTriggerStay2D() was getting called at very specific intervals, and those didn't always line up with the frame that the spacebar went down. My first thought was to just change GetButtonDown() to GetButton(), which calls every frame that the SpaceBar is down. This fixed most of the issues, except when placing a pizza on the counter, which would create a sort of loop and rapidly pick up and drop the pizza on the counter. Not ideal.
Realizing that there was some sort of framerate mismatch, I decided I just needed to decouple the two methods from each other. I created a new Collider2D variable that I could cache the latest collision in, moved the Input check to Update(), and just stored the collider into my new class variable (I also needed to clear the variable in OnTriggerExit2D(), and add a null check in the input event to prevent potential errors). The results looked something like the following:
Collider2D currentTarget; void Update()
{ // If SpaceBar is pressed, and we have a target if (Input.GetButtonDown("Jump") && currentTarget != null) { CheckCollision(currentTarget); } } void OnTriggerStay2D(Collider2D collision) { currentTarget = collision; } void OnTriggerEnter2D(Collider2D collision) { currentTarget = collision; } private void OnTriggerExit2D(Collider2D collision) { // If the collider we left was our current target, clear that target if (collision == currentTarget) { currentTarget = null; } }
I tested it out and it seemed to be working well. Everything is spaced out reasonably well in the game, so it's rare that the player will intersect more than one collider at a time, and if they do, it should just pick one of them (whatever one had OnTriggerStay2D() called last). The game was working again as I had remembered it, and I was able to publish it back up on my itch.io page. There were definitely some gameplay mechanics that I thought could use a revisit if the game were to be developed further, but I was just looking to restore it to its original state for now. If I had the time and interest though, I would have added expiry to cooked pizzas (so you can't just backlog a bunch on the counter and deliver stale pizzas to customers without a rating ding), and I would have adjusted the customer spawn rate, perhaps adding surges and lulls, and a scaling difficulty over time (similar to what I implemented in my most recent jam game, Potato Panic).
Anyway, that's a brief review of some of the work that needed to be done to update and restore one of my old Unity projects. The game is back up and available now at https://ghotifrye.itch.io/home-is-where-the-pizzas-at (just in case I haven't linked it enough times). If you have the time, give it a try and leave a comment. I'd love to hear what you think about it.
Files
Home is Where the Pizza's At
A pizza game developed for Global Game Jam 2019 (Theme: Home)
Status | Released |
Author | August Connolly |
Genre | Action |
Tags | 2D, Arcade, Global Game Jam, pizza |
Leave a comment
Log in with itch.io to leave a comment.